Ionic 2 Modals
Ionic 2 Modals– Modals are basically pane area that appears above the content area. Modals are important component that can be use in different ways such as – show form to edit information, show login form, registration form etc. It is very easy to create Modals in Ionic 2. Here in this tutorial we are going to explain how you can create modals in Ionic 2. You can also use our online editor to run and see the output of the example.
Ionic 2 Modals Example
ModalController is used to create Modals in Ionic 2 so it should be imported first. Let us create a simple modal in Ionic 2-
- Html Part
- Controller Part
Html Part : demo.html
Ionic 2 Modals Html Part Example:
<ion-header> <ion-navbar> <ion-title>Modals</ion-title> </ion-navbar> </ion-header> <ion-content> <ion-list> <a ion-item (click)="openModal({charNum: 0})"> Open Modal </a> </ion-list> </ion-content> |
Html part contains button which will call function to open modal. Now let define the modal in controller part.
Controller Part: demo.ts
Ionic 2 Modals Simple Example:
import { Component } from '@angular/core'; import { ModalController, Platform, NavParams, ViewController } from 'ionic-angular'; import { ModalContentPage } from './modal'; @Component({ templateUrl: 'demo.html' }) export class DemoPage { constructor(public modalCtrl: ModalController) { } openModal(characterNum) { let modal = this.modalCtrl.create(ModalContentPage,characterNum); modal.present(); } } |
Now let us define the modal component for ModalContentPage.
Modal Controller Part : Modal.ts
Modal.ts Script Part Example:
import { Component } from '@angular/core'; import { ModalController, Platform, NavParams, ViewController } from 'ionic-angular'; @Component({ templateUrl: 'modal.html' }) export class ModalContentPage { character; constructor( public platform: Platform, public params: NavParams, public viewCtrl: ViewController ) { var character = [ { image:'https://www.tutorialsplane.com/wp-content/uploads/2017/02/girl.1361906__180.png', name: 'Kelly', text: 'This is kelly from USA.' } ]; this.character = character[this.params.get('charNum')]; } dismiss() { this.viewCtrl.dismiss(); } } |
Template Part : modal.html
Modal Template Part Example:
<ion-header> <ion-toolbar> <ion-title> Profile </ion-title> <ion-buttons start> <button ion-button (click)="dismiss()"> Close </button> </ion-buttons> </ion-toolbar> </ion-header> <ion-content> <ion-list> <ion-item> <ion-avatar item-left> <img src="{{character.image}}"> </ion-avatar> <h2>{{character.name}}</h2> <p>{{character.text}}</p> </ion-item> </ion-list> </ion-content> |
Add Modal Config in app.module.ts
Add the configuration for ModalContentPage, import { ModalContentPage } from ‘../pages/demo/modal’; and add declaration, entryComponents for ModalContentPage.
app.module.ts Configuration:
import { NgModule, ErrorHandler } from '@angular/core'; import { IonicApp, IonicModule, IonicErrorHandler } from 'ionic-angular'; import { MyApp } from './app.component'; import { DemoPage } from '../pages/demo/demo'; import { ModalContentPage } from '../pages/demo/modal'; @NgModule({ declarations: [ MyApp, DemoPage, ModalContentPage ], imports: [ IonicModule.forRoot(MyApp) ], bootstrap: [IonicApp], entryComponents: [ MyApp, DemoPage, ModalContentPage ], providers: [{provide: ErrorHandler, useClass: IonicErrorHandler}] }) export class AppModule {} |
If you run the above example it will create output something like this –
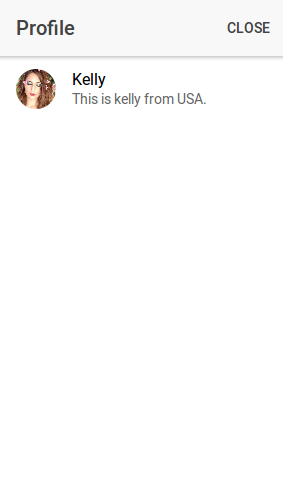
Download Code
file_download Download Sample
Options Available
Instance Methods
Modal Create Method Example:
create(component, data, opts); |
Param | Type | Details |
---|---|---|
component | object | The Modal view |
data | object | Any data to pass to the Modal view |
opts | object | Modal options |
Advanced
Option | Type | Description |
---|---|---|
showBackdrop | boolean | Whether to show the backdrop. Default is true. |
enableBackdropDismiss | boolean | Whether the popover should be dismissed by tapping the backdrop. Default is true. |
ModalCmp ionViewPreLoad error: No component factory found for ModalContentPage
This error comes when you have not created the ModalContentPage module so make sure you have created module correctly.
Advertisements
User Ans/Comments
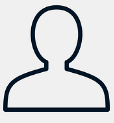
Add Comment
📖 Read More
- 1. Ionic 2 Popover
- 2. Ionic 2 Radio
- 3. Ionic 2 Range
- 4. Ionic 2 Searchbar
- 5. Ionic 2 Segment