Angular Material Dialog
Angular Material Dialog: It is very simple to create a dialog box in Angular Material. <md-dialog> Directive and $mdDialog Service is used to create Dialogs in Angular Material. Here in this tutorial we are going to explain how to create dialog box such as – Alert Box,Confirm Box, Prompt Box, Custom Dialog and Tab Dialog using this directive. You can also use our online editor to edit and run the code online.
Angular Material Dialog Example
Let us first create very basic dialog box in Angular Material-
Angular Material Dialog Box: Alert Box Example
<html lang="en" > <head> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/angular-material/1.1.1/angular-material.min.css"> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular.min.js"></script> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular-animate.min.js"></script> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular-aria.min.js"></script> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular-messages.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/angular-material/1.1.1/angular-material.min.js"></script> <link rel="stylesheet" href="https://fonts.googleapis.com/icon?family=Material+Icons"> <link rel="stylesheet" href="https://fonts.googleapis.com/css?family=Roboto:300,400,500,700,400italic"> <script language="javascript"> angular .module('myApp', ['ngMaterial']) .controller('MyController', function ($scope, $mdDialog) { //controller code goes here... $scope.showAlert = function(ev) { // basic ALert Box $mdDialog.show( $mdDialog.alert() .title('Alert Box Title') .textContent('Your Description text goes here.....') .ariaLabel('Alert Dialog label') .ok('Ok Got it!') .targetEvent(ev) ); }; }); </script> </head> <body ng-app="myApp"> <div ng-controller="MyController" ng-cloak="" > <md-content layout-padding layout="row"> <md-button class="md-primary md-raised" ng-click="showAlert($event)" > Alert Box Dialog </md-button> </md-content> </div> </body> </html> |
Try It On → |
![]() |
![]() |
In the above example we have triggered alert box using JavaScript which uses default template, You can also use custom template.
If you run the above example it will produce output something like this –
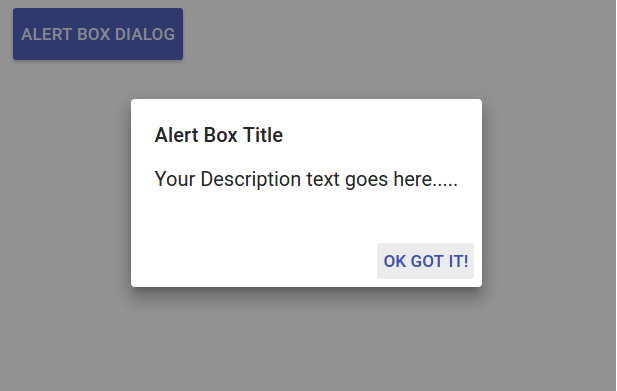
Learn More About Dialog | Popup Box
Let us have some example and demo about the Angular Material Popup box.
Confirm Box
Here is javaScript part which contains the function for confirm box. Just call function showConfirmBox to trigger the confirm popup.You can create confirm box simply as below –
Confirm Dialog Box | Confirm Popup Example:
<script language="javascript"> angular .module('myApp', ['ngMaterial']) .controller('MyController', function ($scope, $mdDialog) { //controller code goes here... $scope.showConfirmBox = function(ev) { var confirm = $mdDialog.confirm() .title('Are you sure to remove this item?') .textContent('It will delete all items permanently.') .ariaLabel('Confirm') .targetEvent(ev) .ok('Yes Delete') .cancel('Cancels'); $mdDialog.show(confirm).then(function() { $scope.status = 'Item Has been Deleted.'; }, function() { $scope.status = 'Canceled.'; }); }; }); </script> |
Try It On → |
![]() |
![]() |
If you run the above example it will produce output something like this –
Prompt Dialog Box
JavaScript Part for prompt box is as below-
Prompt Dialog Box Example:
<script language="javascript"> angular .module('myApp', ['ngMaterial']) .controller('MyController', function ($scope, $mdDialog) { //controller code goes here... $scope.showPromptBox = function(ev) { var confirm = $mdDialog.prompt() .title('Enter A username?') .textContent('Add a unique username.') .placeholder('Username') .ariaLabel('Username') .initialValue('john123') .targetEvent(ev) .ok('Okay!') .cancel('Cancel'); $mdDialog.show(confirm).then(function(result) { $scope.status = 'Your username saved as ' + result + '.'; }, function() { $scope.status = 'You cancelled the action.'; }); }; }); </script> |
Try It On → |
![]() |
![]() |
If you run the above example it will produce the following output –
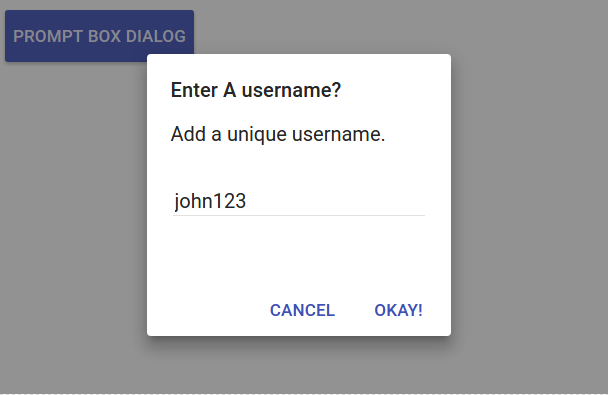
Pre-Rendered Dialog | Modal Example
Sometimes we need pre-rendered dialog rather than adding the content from Script. It pre-rendered dialog template is not executed each time. It is very simple to create pre-rendered dialog. Here is an example of creating pre-rendered dialog.
Pre-Rendered Dialog Box | Modal Example
<html lang="en" > <head> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/angular-material/1.1.1/angular-material.min.css"> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular.min.js"></script> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular-animate.min.js"></script> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular-aria.min.js"></script> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular-messages.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/angular-material/1.1.1/angular-material.min.js"></script> <link rel="stylesheet" href="https://fonts.googleapis.com/icon?family=Material+Icons"> <link rel="stylesheet" href="https://fonts.googleapis.com/css?family=Roboto:300,400,500,700,400italic"> <script language="javascript"> angular .module('myApp', ['ngMaterial']) .controller('MyController', function ($scope, $mdDialog) { //controller code goes here... $scope.showPrerenderedDialogBox = function(ev) { $mdDialog.show({ controller: dialogCtr, contentElement: '#myDialogBox', parent: angular.element(document.body), targetEvent: ev, clickOutsideToClose: true }); }; function dialogCtr($scope, $mdDialog) { $scope.hide = function() { $mdDialog.hide(); }; $scope.cancel = function() { $mdDialog.cancel(); }; $scope.answer = function(answer) { $mdDialog.hide(answer); }; } }); </script> </head> <body ng-app="myApp"> <div ng-controller="MyController" ng-cloak="" > <md-content layout-padding layout="row"> <md-button class="md-primary md-raised" ng-click="showPrerenderedDialogBox($event)" > Show Pre Rendered Dialog </md-button> <div style="visibility: hidden"> <div class="md-dialog-container" id="myDialogBox"> <md-dialog layout-padding> <h2>Pre-Rendered Dialog Box Example</h2> <p> Content goes..... here. </p> </md-dialog> </div> </div> </md-content> </div> </body> </html> |
Try It On → |
![]() |
![]() |
Advertisements