KnockoutJS Observable Array
KnockoutJS Observable Array – KnockoutJs provides Observable Array(observableArray) to detect and respond to changes in a collection of things. Sometimes we need to reflect the changes to UI when working with multiple values like adding new values, editing new values or deleting the values. Here in this tutorial we are going to explain how you can use Observable Array in KnockoutJS with example and demo.
KnockoutJS Observable Array Example
Let us understand how Observable Array works-
Syntax
You can initialize empty array simple as below-
KnockoutJS Observable Array Example:
var myObservableArray = ko.observableArray(); // Initialize empty array |
Example
Now let us create a simple example of observableArray
Observable Array Example:
<!DOCTYPE html> <head> <title>KnockoutJS Example</title> <script src="https://cdnjs.cloudflare.com/ajax/libs/knockout/3.3.0/knockout-min.js" type="text/javascript"></script> </head> <body> <div> <form data-bind="submit: addItem"> New item: <input data-bind='value: itemToAdd, valueUpdate: "afterkeydown"' /> <button type="submit" data-bind="enable: itemToAdd().length > 0">Add</button> <p>Your items:</p> <select multiple="multiple" width="50" data-bind="options: myObservableArray"> </select> </form> </div> <script> // data model content goes here var ViewModel = function() { this.myObservableArray = ko.observableArray(['Mango', 'Banana', 'Apple']); // Initialize an array this.itemToAdd = ko.observable('Test');// Add some default value to input this.addItem = function(){ if(this.itemToAdd().length > 0){ this.myObservableArray.push(this.itemToAdd()); } }; }; // Activate Knockout.JS ko.applyBindings(new ViewModel()); </script> </body> </html> |
If you run the above example it will produce the output something like this-
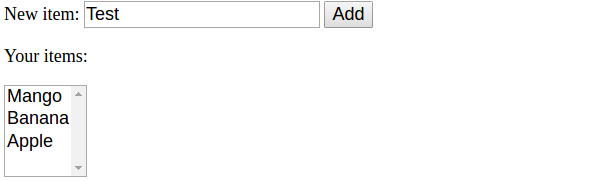
Define Array Objects
You can also define objects as an observableArray simply as below –
Observable Array Objects Example:
var observableArray = ko.observableArray([ { name: "John", country: "USA" }, { name: "Kelly", country: "Japan" }, { name: "Rimmy", country: "Poland" } ]); |
Get Observable Array Length
You can find the Obseravble Array Length as below-
Observable Array Get Length Example:
var observableArray = ko.observableArray([ { name: "John", country: "USA" }, { name: "Kelly", country: "Japan" }, { name: "Rimmy", country: "Poland" } ]); var obseravleArrayLength = observableArray.lentgh; |
Learn More
Observable Array indexOf
indexOf function returns the indexOf the array item. If item is found in array it will return value > -1 else it will return -1.
Observable Array Manupulation
There are following methods available to perform the Array Manupulation-
- push(value)– This method adds a new item at the end of array. Example –
var myObservableArray = ko.observableArray(); myObservableArray.push('Some value');
- pop()– This removes the last item from the array.
- unshift( value )– This inserts a new value at the begining of the array.
- shift()– It removes the first value from array and returns it.
- reverse()– This reverses the array items and retruns it.
- sort()– Sorts the array contents and returns the observableArray.
- splice()– Removes and returns a given number of elements starting from a given index. Example-
myObservableArray.splice(1, 3);
Removes the elements from index 1 to index 3 and returns the ObservableArray.
- remove(someItem)– Removes the given item from observableArray and returns observableArray.
- remove( function (item) { return item.age < 18; } ) –
If you want to remove the items based upon some condition then you can use the function as above. - removeAll()– Removes all items from array.
- removeAll([‘item1’, 123, undefined])– This will remove the specified items only ie- ‘item1’, 123, undefined and return array.
- –
- –
Advertisements
User Ans/Comments
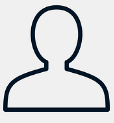