KnockoutJS Computed Observables
KnockoutJS Computed Observables– Computed observables are functions that are dependent upon one or more observables, computed observables automatically update if any of them change. Here in this tutorial, we are going to explain how you can create computed observables and use them. You can use our online editor to edit and run the code online.
KnockoutJS Computed Observables Example
Syntax
Sntax of Computed Observables is-
KnockoutJS Computed Observables Example:
this.variableName = ko.computed(function() { // your code goes here.. }, this); |
Let us create a very basic example to understand the Computed Observables –
KnockoutJS Computed Observables Example:
<!DOCTYPE html> <head> <title>KnockoutJS Example</title> <script src="https://cdnjs.cloudflare.com/ajax/libs/knockout/3.3.0/knockout-min.js" type="text/javascript"></script> </head> <body> <div> FirstName <input data-bind='value: firstname' /><br> LastName <input data-bind='value: lastname' /><br> Hello <span data-bind="text:fullname">. </div> <script> // data model content goes here var ViewModel = function() { this.firstname = ko.observable('Dave'); this.lastname = ko.observable('Smith'); this.fullname = ko.computed(function() { return this.firstname() + " " + this.lastname(); }, this); }; // Activate Knockout.JS ko.applyBindings(new ViewModel()); </script> </body> </html> |
So you can if you change in any input field it will automatically update fullname, thus you handle more than one observable variables using the Computed Variables.
If you run the above example it will produce the output something like this-

Pure Computed Observables
Pure Computed Observables were introduced in KnockoutJs 3.2.0 to provide the memory and performance over the regular Computed Observables. Pure computed observables does not maintain the subscriptions to its dependencies when it has no subscriptions.
Here are main features of Pure Computed Observables-
- 1. Prevents Memory Leakage– It prevents memory leakage from the observables that are no longer referenced in the application but their dependencies still exists.
- 2. Reduces computation overhead– It reduces overhead by ignoring the observable whose value is not being observed.
Syntax
Syntax of pure observables is as –
Pure Computed Observables Example:
this.variableName = ko.pureComputed(function() { // your code goes here.. }, this); |
Pure Computed Observable Example
Let us create an example of Computed Observables-
Pure Computed Observables Example:
<!DOCTYPE html> <head> <title>KnockoutJS Example</title> <script src="https://cdnjs.cloudflare.com/ajax/libs/knockout/3.3.0/knockout-min.js" type="text/javascript"></script> </head> <body> <div> FirstName <input data-bind='value: firstname' /><br> LastName <input data-bind='value: lastname' /><br> Hello <span data-bind="text:fullname">. </div> <script> // data model content goes here var ViewModel = function() { this.firstname = ko.observable('Dave'); this.lastname = ko.observable('Smith'); this.fullname = ko.pureComputed(function() { return this.firstname() + " " + this.lastname(); }, this); }; // Activate Knockout.JS ko.applyBindings(new ViewModel()); </script> </body> </html> |
Writable Computed Observables
If you have noticed the regular Computed Observables, they are read only ie. you can’t modify the observables value. Writable computed Observables enable us to create computed observables whose value can be modified(writable).
Let us create a simple example of Writable Computed Observables-
Writable Computed Observables Example:
<!DOCTYPE html> <head> <title>KnockoutJS Example</title> <script src="https://cdnjs.cloudflare.com/ajax/libs/knockout/3.3.0/knockout-min.js" type="text/javascript"></script> </head> <body> <div> FirstName <input data-bind='value: firstname' /><br> LastName <input data-bind='value: lastname' /><br> Hello <span data-bind="text:fullname">. </div> <script> // data model content goes here var ViewModel = function() { this.firstname = ko.observable('Dave'); this.lastname = ko.observable('Smith'); this.fullname = ko.pureComputed({ read: function () { return this.firstname() + " " + this.lastname(); }, write: function (value) { this.firstname(value.substring(0, 3)); // Update "firstName" this.lastname(value.substring(0, 2)); // Update "lastName" }, owner: this }, this); }; // Activate Knockout.JS ko.applyBindings(new ViewModel()); </script> </body> </html> |
Advertisements
User Ans/Comments
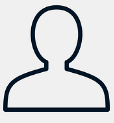