Category Archives: KnockoutJS Tutorial
KnockoutJS Computed Observables
KnockoutJS Computed Observablesβ Computed observables are functions that are dependent upon one or more observables, computed observables automatically update if any of them change. Here in this tutorial, we are going to explain how you can create computed observables and use them. You can use our online editor to edit and run the code online.
KnockoutJS Computed Observables Example
Syntax
Sntax of Computed Observables is-
KnockoutJS Computed Observables Example:
this.variableName = ko.computed(function() { // your code goes here.. }, this); |
Let us create a very basic example to understand the Computed Observables β
So you can if you change in any input field it will automatically update fullname, thus you handle more than one observable variables using the Computed Variables.
If you run the above example it will produce the output something like this-

Pure Computed Observables
Pure Computed Observables were introduced in KnockoutJs 3.2.0 to provide the memory and performance over the regular Computed Observables. Pure computed observables does not maintain the subscriptions to its dependencies when it has no subscriptions.
Here are main features of Pure Computed Observables-
- 1. Prevents Memory Leakageβ It prevents memory leakage from the observables that are no longer referenced in the application but their dependencies still exists.
- 2. Reduces computation overheadβ It reduces overhead by ignoring the observable whose value is not being observed.
Syntax
Syntax of pure observables is as β
Pure Computed Observables Example:
this.variableName = ko.pureComputed(function() { // your code goes here.. }, this); |
Pure Computed Observable Example
Let us create an example of Computed Observables-
Pure Computed Observables Example:
|
Writable Computed Observables
If you have noticed the regular Computed Observables, they are read only ie. you canβt modify the observables value. Writable computed Observables enable us to create computed observables whose value can be modified(writable).
Let us create a simple example of Writable Computed Observables-
Writable Computed Observables Example:
|
KnockoutJS Observable Array
KnockoutJS Observable Array β KnockoutJs provides Observable Array(observableArray) to detect and respond to changes in a collection of things. Sometimes we need to reflect the changes to UI when working with multiple values like adding new values, editing new values or deleting the values. Here in this tutorial we are going to explain how you can use Observable Array in KnockoutJS with example and demo.
KnockoutJS Observable Array Example
Let us understand how Observable Array works-
Syntax
You can initialize empty array simple as below-
KnockoutJS Observable Array Example:
var myObservableArray = ko.observableArray(); // Initialize empty array |
Example
Now let us create a simple example of observableArray
If you run the above example it will produce the output something like this-
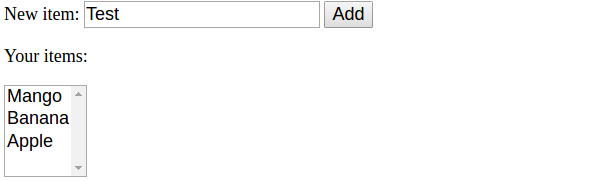
Define Array Objects
You can also define objects as an observableArray simply as below β
Observable Array Objects Example:
var observableArray = ko.observableArray([ { name: "John", country: "USA" }, { name: "Kelly", country: "Japan" }, { name: "Rimmy", country: "Poland" } ]); |
Get Observable Array Length
You can find the Obseravble Array Length as below-
Observable Array Get Length Example:
var observableArray = ko.observableArray([ { name: "John", country: "USA" }, { name: "Kelly", country: "Japan" }, { name: "Rimmy", country: "Poland" } ]); var obseravleArrayLength = observableArray.lentgh; |
Learn More
Observable Array indexOf
indexOf function returns the indexOf the array item. If item is found in array it will return value > -1 else it will return -1.
Observable Array Manupulation
There are following methods available to perform the Array Manupulation-
- push(value)β This method adds a new item at the end of array. Example β
var myObservableArray = ko.observableArray(); myObservableArray.push('Some value');
- pop()β This removes the last item from the array.
- unshift( value )β This inserts a new value at the begining of the array.
- shift()β It removes the first value from array and returns it.
- reverse()β This reverses the array items and retruns it.
- sort()β Sorts the array contents and returns the observableArray.
- splice()β Removes and returns a given number of elements starting from a given index. Example-
myObservableArray.splice(1, 3);
Removes the elements from index 1 to index 3 and returns the ObservableArray.
- remove(someItem)β Removes the given item from observableArray and returns observableArray.
- remove( function (item) { return item.age < 18; } ) β
If you want to remove the items based upon some condition then you can use the function as above. - removeAll()β Removes all items from array.
- removeAll([βitem1β, 123, undefined])β This will remove the specified items only ie- βitem1β, 123, undefined and return array.
- β
- β
KnockoutJS Observables
KnockoutJS Observables Observables are special JavaScript objects that are used widely in KnockoutJS to handle the data exchange between view-model and view. Here in this tutorial we are going to explain how observables works in KnockoutJS.
KnockoutJS Observables | Syntax | Example
KnockoutJS basically works on the following three features-
- 1.Observables and dependency tracking β Observables plays an important in data exchange between View and View-Model. Observables takes care of dependency tracking.
- 2.Declarative bindingsβ data-bind is used in view to handle the data(update) in ViewModel.
- 3.Templatingβ KnockoutJs supports templating which enables us to create rich applications.
Syntax
KnockoutJS Observables Syntax:
this.propertyName = ko.observable('value'); |
You can just make any property observable. ko is globally available once you include the KnockouJS library. βvalueβ will be assigned to the view model property ie. to propertyName.
Example
Now let us create very basic example to understand the observables-
If you run the above the example it will produce the output something like this-
If you change the input box value it will automatically update the view part.

Activate KnockoutJS
As we know data-bind is not predefined HTML attribute so HTML will not understand the data-bind meaning so it is necessary to activate it. You can activate KnockoutJS simply as below-
| Example:
// Activate Knockout.JS ko.applyBindings(new ViewModelName()); |
Where ViewModelName is model that you want to activate.
Observables Read And Write
- 1. Readβ You can read the model property by just β myViewModel.propertyName(); Example myViewModel.personName() β
- 2. Writeβ To write value you just need to pass value as parameter as β myViewModel.personName(βKellyβ).
- 2. Write Multiple Valuesβ You can also write multiple values at a time simply as β myViewModel.personName(βKellyβ).personAge(30).personCity(βNewYorkβ).
KnockoutJS Architecture
KnockoutJS Architectureβ KnockoutJS is specially designed to create Single Page web applications. It provides functionality for loading data without reloading or navigating to the different page. It refreshes only the required part of UI.
As we all know nowadays mobile devices have become the primary device to access the web(internet), websites are known to load slowly on mobile devices but now there several technologies(AngularJs, KnockoutJS) which enable us to develop websites with great performance.
KnockoutJS Architechture
It is JavaScript Framework which enables us to develop web apps with great performance and works on all devices.
KnockoutJs is basically follows the Model-View-ViewModel(MVVM) pattern.
Now let us understand what is Model-View-ViewModel.
1. Model
Model basically handles the request & response between server & ViewModel. It communicates to the data server, It listens the request from the ViewModel and sends it to the server and provides the response back to viewModel.
2. ViewModel
ViewModel basically holds the data objects returned from the model and directly communicates to the view. It is responsible to change the data in view, it automatically refreshes the Ui if there is change in data. It uses observable to watch the changes.
3. View
View is the part which is directly visible to the users. data-bind is used to handle the data in View part which automatically updates the changes in UI.
KnockoutJS First Program
KnockoutJS First Program- Let us first create a very basic and simple program in KnockoutJs to understand how it works.
KnockoutJS First Program | Hello World Example
In this tutorial we are going to create a simple example which contains two input fields- Firstname and Lastname. We will print the input box values using KnockoutJs. This example has two parts simply as below β
- 1. View β This contains the html UI parts which will be updated frequently as soon as the data model values are changed.
- 2. View Model β This contains the data model part, this sits behind UI layer and exposes data needed by a View.
View
View Part contains the following code-
KnockoutJs First Example: View
|
View Model
View Model Part contains the following code-
KnockoutJs First Example: View Model
|
Complete Example
Now let us combine the both parts and create the full example as below β
If you run the above example it will produce output something like this-
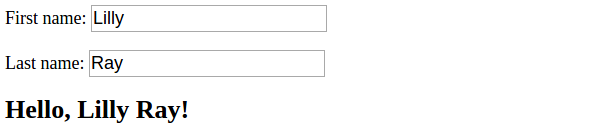
KnockoutJS Environment Setup
KnockoutJS Environment Setup It is very easy to install KnockoutJs, You can download the library and install it locally or you can use the hosted CDN. Here in this tutorial, we are going to explain the both ways to install the KnockoutJS.
KnockoutJS Environment Setup | Installation Setps
You can install KnockoutJS in following ways-
- 1. Download and Install Locally β
- 2. Use Hosted CDNβ
1 Download And Install Locally
You can download the latest production build of KnockoutJs from itβs official site β Download KnockoutJs
After Downloading just keep the download js file in some folder(such as js) and include it simply as below-
KnockoutJS Install locally Example:
|
After Including this file you will be able to run the KnockoutJS programs.
2. Use Hosted CDN | KnockoutJS CDN Url
If you do not want to download the KnockoutJS library you use the Hosted CDN urls to include the library in your web application, There are following hosted CDN providers for KnockoutJs β
KnockoutJS Cloudflare CDN Url
KnockoutJS Cloudflare CDN Url:
|
KnockoutJS MicroSoft Ajax CDN Url
KnockoutJS Microsoft Ajax CDN Url:
|
After installing this we are able to create the first example.
KnockoutJS Tutorial
KnockoutJS Tutorial: KnockoutJS is JavaScript Library which enables us to create rich, responsive applications. KnockoutJS is based on MVVM(Model, View, View Model) architecture. KnockoutJS helps you to implement the UI update functionality that is changing dynamically based on the user interaction or external data source change. KnockoutJS is very simple to learn.
Here in this tutorial, we are going to cover the basic and advanced topics in KnockoutJS.
KnockoutJS Tutorial With Example & Demo
Here in this tutorial we will explain each topic with example and demo.
KnockoutJs Features
KnockoutJs has following main features-
- Elegant dependency tracking: KnockoutJs updates only the right(required) parts of the UI when there is change in data model.
- Declarative bindings: A easiest way to connect the UI parts to the data model.
- Trivially extensible: You can implement custom behaviors as new declarative bindings for reusing the code.
- Templating: It Provides templating functionality to develop complex UI.
KnockoutJs Benefits
KnockoutJs has following benefits which makes it different β
- Pure JavaScript Library: It is pure JavaScript Library which works with any client or server side language.
- Compact: It is very light weight library which is just 13KB after gzip.
- Supports on All Browsers: It works almost on all mainstream browsers.
You Should Know
Before starting you should be aware of HTML, CSS, JavaScript and Document object model(DOM).