Category Archives: Vue.Js Blog
Vue.js Event Watch Route Change
We can use watch property to track the change in route change. It is very simple to use Watch Route change in vue.js. Here in this article we are going to explain how you can get the route change in Vue.js.
Vue.js Event Watch Route Change Example
You can use watch property simply as below –
Example:
|
On the same way you can track the change in Router(URL).
Vue.Js Remove Hash From URL
It is super simple to remove hashbang(#) from URL in vuejs. You can follow Below Steps to remove Hash from URL.
Vue.Js Remove Hash From URL | Router Example
You just need to set mode: ‘history’ in Vue Router Configuration. Here is simple Change you need to do-
Example:
|
Unknown custom element: vue.js
Here is working example. I was missing Vue.use(VueRouter); I added this and it worked for me.
import Vue from 'vue' import App from './App.vue' import VueRouter from 'vue-router' import HelloWorld from './components/HelloWorld.vue' import LoginBlock from './components/LoginBlock.vue' Vue.config.productionTip = false const routes = [ { path: '/foo', component: HelloWorld }, { path: '/bar', component: LoginBlock } ] const router = new VueRouter({ routes // short for `routes: routes` }) Vue.use(VueRouter); new Vue({ router, render: h => h(App), }).$mount('#app')
Uncaught TypeError: router.map is not a function vue.js
The function .map is deprecated in Latest Version of Vue.js Use the below instead of map –
<pre>
new VueRouter({
routes: [
{ path: ‘/foo’, component: LoginBlock }
],
});
</pre>
For router the above example will work perfectly.
Vue.js Local Storage
Vue.js Local Storage– We can use the native javascript localstorage in Vue.js.JavaScript localStorage property allows us to save data in localstorage. Here in this tutorial, we are going to explain how you can use localstorage property to save data on client side. You can also use our online editor to edit and run the code online.
Vue.js Local Storage Example
Let us understand how localStorage works in JavaScript and how we can use it in vuejs. –
Syntax
Here is syntax of storage object-
var currStorage = window.localStorage;
This is used to access the current origin’s local storage space.
Set Item
You can set Item data in local storage simply as below-
localStorage.setItem('myItem', "Hello World!")
Get Item
You can read data from local storage simply as below-
var myData = localStorage.getItem('myItem');
Remove Item From LocalStorage
You can remove data from local storage simply as below-
localStorage.removeItem('myItem');
localStorage has no expiration time.
For Web Storage Api Details You can get more details here – Web Storage API Documentaion
Now let us create an example in vue.js.
Example:
|
Output of above example-
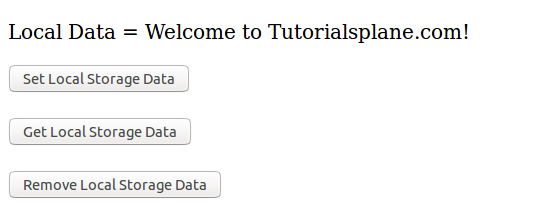
Vue.js Display image
Vue.js Display image We can use v-bind:src=”” to display image in vue.js. We can also use :src=”” shorthand to show image in vuejs. Here in this article we are going to explain how you can display image in vuejs. You can also use our online editor to edit and run the code online.
Vue.js Display image Example
Here is simple example to –
In the above example we have create myFucntion to assign image src to variable myImage. When you click on the show image button it will update the variable and display image.
Output of above example-
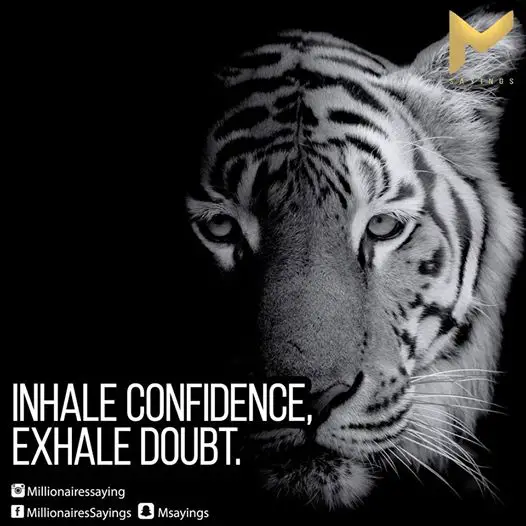
Vue.js JSON Stringify
We can use native JavaScript JSON stringify() method to convert json object to json string. Here in this tutorial, we are going to explain how you can use this method to convert an json object to json string in JavaScript in vuejs. You can also use our online editor to edit and run the code online.
Vue.js JSON stringify – JavaScript Example
You can use stringify() method in vuejs simply as below-
Output of above example-
Vue.js Parse JSON String
We can use native JavaScript parse() method to parse json string. Here in this tutorial, we are going to explain how you can use this method to parse a string in JavaScript Object in vuejs. You can also use our online editor to edit and run the code online.
Vue.js Parse JSON String – JavaScript Example
You can use parse() method in vuejs simply as below-
Output of above example-
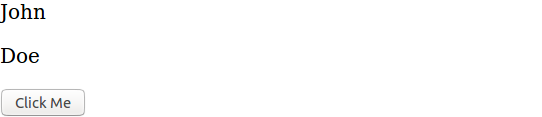
Vue.js Get Screen Width
We can use screen width property to get the total width of the device screen. Here in this tutorial, we are going to explain how you can use screen width object to get the width of the screen. You can also use our online editor to edit and run the code online.
Vue.js get screen Width Example
You can get device screen width in vuejs simply as below-
Output of above example-
