Category Archives: AngularJs
AngularJs Forms
AngularJs Forms : An AngularJs Forms contains the input fields known as controls. Controls are used by user to enter the data.
AngularJs Forms example
AngularJs Modules
AngularJs Modules : AngularJs Modules works as container for controllers. Controllers are associated with modules.
Example for AngularJs Modules
Let us understand with the following example.
We have created an application named as “myApp” which have the controller named as “myController”.
Name :
Name :
Name : {{ name }}
Email : {{ email }}
Now let us create module
AngularJs Modules Full Example
Output :
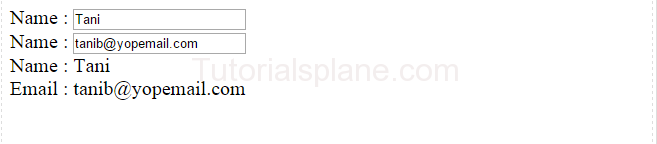
AngularJs Modules
Note : You can keep your module and controller javaScript code in external js file. Example module.js and controller.js. Include these file such as
Example
myApp.js will have following code.
var myApp = angular.module("myApp", []);
myController.js will have following code.
myApp.controller("myController", function($scope) { $scope.name = "Tani"; $scope.email = "tanib@yopemail.com"; });
AngularJs Events
AngularJs Events : There are event directives available in AngularJs. Example : ng-click, ng-show, ng-hide etc.
List of AngularJs Events
Following events are available in angularJs directive.
[table width=”100%” colwidth=”10|100|290|160″ colalign=”left|left|left|left|left”]
No,Events ,Description, Example & Detail, Demo
1.,ng-click,Binds the click event, More Detail & Demo », Demo »
2.,ng-dblclick,Binds the double click event, More Detail & Demo », Demo »
3.,ng-mousedown,Binds the mousedown event, More Detail & Demo », Demo »
4.,ng-mouseenter,Binds the mouseenter event, More Detail & Demo », Demo »
5.,ng-mouseleave,Binds the mouseleave event, More Detail & Demo », Demo »
6.,ng-mousemove,Binds the mousemove event, More Detail & Demo », Demo »
7.,ng-keydown,Binds the keydown event, More Detail & Demo », Demo »
8.,ng-keyup,Binds the keyup event, More Detail & Demo », Demo »
9.,ng-keypress,Binds the keypress event, More Detail & Demo », Demo »
10.,ng-change,Binds the change event, More Detail & Demo », Demo »
[/table]
AngularJs DOM
AngularJs DOM : AngularJs provides directives which binds application data with the HTML attributes.
AngularJs DOM Directives
[table width=”100%” colwidth=”10|100|290|160″ colalign=”left|left|left|left|left”]
No,Filters ,Description, Example & Detail, Demo
1.,ng-disabled,Disables the Element, More Detail & Demo », Demo »
2.,ng-show,Shows the hidden Element, More Detail & Demo », Demo »
3.,ng-hide,Hides the Element, More Detail & Demo », Demo »
3.,ng-click,Binds the click event, More Detail & Demo », Demo »
[/table]
AngularJs Tables
AngularJs Tables : Tables in AgularJs are created using the ng-repeat directives. You can create tables and add
css styles to them.
AngularJs Tables : Syntax
Example
......
|
Add Css In Table
You can add css directly in table tr and td tags or you can add it by adding custom classes.
AngularJs $even and $odd Example
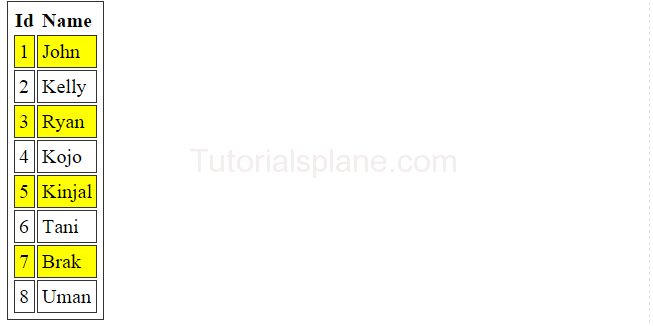
AngularJs Tables
Angularjs Http Post Method
Angularjs Http Post Method : Angularjs Http Post is used to perform post request. It posts data using post method. This method is preferred when you are working with the data which is more secure or you are sending large data. We are going to explain post method with the example and demo.
Angularjs Http Post Method Syntax | Config | Example | Demo
Syntax for Post method –
Angularjs $http.post Syntax:
$http.post(url, data, [config]).then(Callback); |
Parameters
- url : Thet Url from where you want to load data.
- data : data you want to post.
- config(optional): This is optional configuration object .
- Callback : Callback function after success. Returns object.
Returns
- Returns : HttpPromise Object.
Angularjs Http Post Method : Php File
Here we are using php at server side to save data. We will post data on the postUsers.php file and save it in table.
Angularjs $http.post Example :
$postdata = file_get_contents("php://input"); $request = json_decode($postdata); $name = addslashes($request->name); $email = addslashes($request->email); $phone = addslashes($request->phone); $message = ''; if(!empty($name)&& !empty($email)){ $query = mysql_query("insert into test_user (name,email,phone)values('$name','$email','$phone')"); } |
Here is an example of post method in which we are posting posting data using post method. To see the output of the example click on “try it” button –
Angularjs $http.post example with parameters | JSON
|
The above example will produce the following output of the form to view full functionality click on “Try it” button and fill form and submit to post and see result –
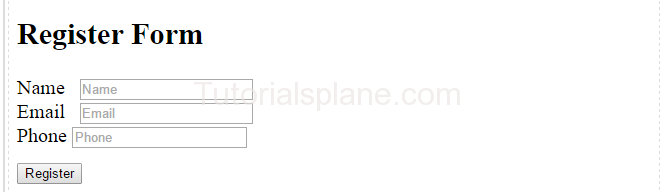
Angularjs Http Post Method Example
Angularjs $http post multiple parameters –
If you want to send the multiple parameters using $http post method you can pass multiple parameters as below –
$http.post("url",{'param1': value1,'param2': value2,'param3': value3,'param4': value4})
More About $http Post Method
Let’s have look over more about $http post method.
Headers
The $http service automatically adds some HTTP headers to each requests. You can configure the default headers by accessing the $httpProvider.defaults.headers configuration object. $httpProvider.defaults.headers contains the current header configuration.
-
$httpProvider.defaults.headers.common (Common Headers for all requests):
Accept: application/json, text/plain, * / *
-
$httpProvider.defaults.headers.post: ( Default Headers for POST requests)Content-Type: application/json
-
$httpProvider.defaults.headers.put (Default Headers for PUT requests)Content-Type: application/json
You can add or overwrite the defaults simply adding a new object with the lowercase HTTP method name as the key, e.g. $httpProvider.defaults.headers.get = { ‘Test-Header’ : ‘value’ }.
Angularjs http post set Header-
You can set content type header in angularjs $http post method simply as below –
Angularjs Set http post content type json-
Angularjs Set http post content type json Example :
var req = { method: 'POST', url: 'http://tutorialsplane.com/runtest/json/registerUsers.php', headers: { 'Content-Type': 'application/json' }, data: {'name': $scope.name,'email': $scope.email,'phone': $scope.phone} } $http(req).success(function(response) {$scope.usersData = response.users;$scope.message = response.message;}); |
Try Video Demo – All In One Video for this method –
AngularJs Http Post Method Video Demo –
Angularjs Http Get Method
Angularjs Http Get Method : AngularJs Http Get is used to perform get request. The $http is basically core angular service that provides communication with remote HTTP services via the XMLhttpRequest object or via JSONP. You can use this method to communicate with sever using the get method. We are going to cover this method with example and demo.
Angularjs Http Get Method Syntax
Here is syntax of Http get method –
$http.get('Url').then(Callback);
Url : Thet Url from where you want to load data.
Callback : Callback function after success. Returns object.
Angularjs Http Get Method Sample Data
Sample data for http get method is given below which is used in get method example.
{"users":[{"id":"1","name":"John","email":"johnk@yopemail.com","phone":"121323232"},{"id":"2","name":"Kelly","email":"kellyk@yopemail.com","phone":"121212122"},{"id":"3","name":"Ryan","email":"ryank@yopemail.com","phone":"22222212"},{"id":"4","name":"Kojo","email":"kojao@yopemail.com","phone":"12144444"},{"id":"5","name":"Kinjal","email":"kinjal@yopemail.com","phone":"22555212"},{"id":"6","name":"Tani","email":"tanya@yopemail.com","phone":"121334444"},{"id":"7","name":"Brak","email":"barak@yopemail.com","phone":"2444444512"},{"id":"8","name":"Uman","email":"uman@yopemail.com","phone":"12334444"}]}
Below is example of Http Get method which reads data in JSON format from the url : http://runtest.tutorialsplane.com/json/getUsers.php. You can use your server path which will return data in JSON format. Run the below example to see output.
If you run the above example it will produce output like this-
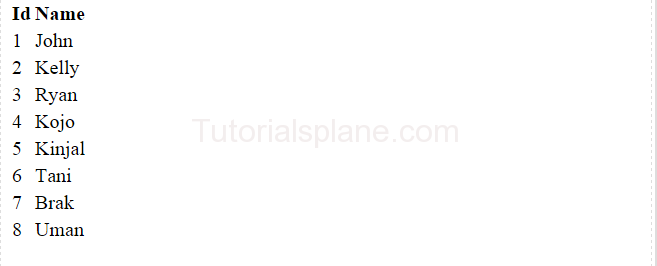
Angularjs Http Get Method
Try Video Demo – All In One Video for this method –
AngularJs Http Get Method Video Demo –
Angularjs Http Intro
Angularjs Http Intro : AngularJs Http is core service used to read data from remote server. It basically interacts with HTTP servers and reads data.
Syntax : Angularjs Http Intro
$http.get('Url').then(Callback); $http.post('Url', data).then(Callback);
Url : Thet Url from where you want to load data.
Callback : Callback function after success.
Let us call getUsers.php from server with the following data
{"users":[{"id":"1","name":"John","email":"johnk@yopemail.com","phone":"121323232"},{"id":"2","name":"Kelly","email":"kellyk@yopemail.com","phone":"121212122"},{"id":"3","name":"Ryan","email":"ryank@yopemail.com","phone":"22222212"},{"id":"4","name":"Kojo","email":"kojao@yopemail.com","phone":"12144444"},{"id":"5","name":"Kinjal","email":"kinjal@yopemail.com","phone":"22555212"},{"id":"6","name":"Tani","email":"tanya@yopemail.com","phone":"121334444"},{"id":"7","name":"Brak","email":"barak@yopemail.com","phone":"2444444512"},{"id":"8","name":"Uman","email":"uman@yopemail.com","phone":"12334444"}]}
Angularjs Http Intro : Example
AngularJs Filters
AngularJs Filters :Provides some filters which are applied before displaying the data. Pipe line Character is used to apply the filters
AngularJs Filters : Following types of Filters are available
- filter– Filter the data based on criteria.
- uppercase-Converts in uppercase
- lowercase-Converts in lowercase
- currency-Converts in currency
- orderby-Sorts array data based on criteria
[table width=”100%” colwidth=”10|100|290|160″ colalign=”left|left|left|left|left”]
No,Filters ,Description, Example & Detail, Demo
1.,filter,Filter the data based on criteria, More Detail & Demo », Demo »
2.,uppercase,Converts in uppercase, More Detail & Demo », Demo »
3.,lowercase,Converts in lowercase, More Detail & Demo », Demo »
3.,Currency,Converts in currency, More Detail & Demo », Demo »
3.,orderby,Sorts array data based on criteria, More Detail & Demo », Demo »
[/table]