Vue.js Remove item from Array
Vue.js Remove item from Array– You can use .splice method to remove an item from array or object. Here in this tutorial, we are going to explain how you can remove items from array. You can also use our online editor to edit and run the code online.
Vue.js Remove item from Array Example
Here we have created simple example to remove items from array or object.
Example:
<!DOCTYPE html> <html> <head> <script src="https://cdnjs.cloudflare.com/ajax/libs/vue/2.5.13/vue.js"></script> </head> <body> <div id="app"> <h1>List</h1> <div v-for="(listItem, index) in list"> <input v-model="listItem.a" value="{{listItem.a}}"> <button @click="deleteList(index)"> Delete List </button> </div> <button @click="addList"> Add New List </button> <b>{{ list }}</b> </div> <script> new Vue({ el: '#app', data: { list: [{a: 'Hello World!' }] }, methods: { addList: function () { this.list.push({ a: 'Hello World!' }); }, deleteList: function (index) { this.list.splice(index, 1); } } }); </script> </body> </html> |
Ouput of above example-
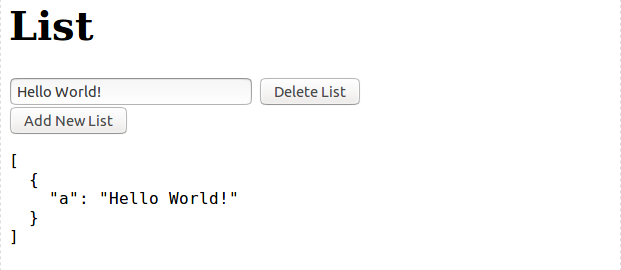
Advertisements