Ionic Popup
Ionic Popup- Popups are basically used to draw user’s attention and get some information from the user or provide some information. Popups Appear on the top of app’s content. You can manually dismiss the popup to interact the app again.
Ionic Provides various popup alerts that can be used easily in any application. There are – Basic Alerts, Prompt Alerts, Confirmation Alerts, Radio Alerts and Checkbox Alerts available in Ionic.
Here in this tutorial, we are going to explain how to create ionic popup alert step by step with example and demo.You can also use our online editor to edit and run the code online.
Note : These popups are supported in Angular Version > 2.x.
Ionic Popup | Alert | Prompt | Confirmation Alert | Example
Popup is dialog box that is used to show important message or information to User or It can can used
to get some input from user for example – Prompt box to enter wi-fi username/password.
Now let us understand popup alert with example & demo –
- 1. Basic Alerts
- 2. Prompt Alerts
- 3. Confirmation Alerts
- 4. Radio Alerts
- 5. Checkbox Alerts
AlertController is required to create alerts so don’t forget to import this controller.
Basic Alerts
- 1. HTML Part – It contains the view part of basic alert.
- 2. Script Part – This contains typescript code for the basic Alert.
Html Part : demo.html
So now add the following code to create button layout-
Ionic Popup Example: Html Part
<ion-content padding> <h2>Alert</h2> <button ion-button block (click)="showAlert()"> Show Alert </button> </ion-content> |
Html contains the Layout part of the page, a button “Show Alert” is created to call function showAlert() which opens a basic alert, this function is defined in JavaScript Typescript – demo.ts file.
Script Part : demo.ts
It contains script resources to create Alert Box, showAlert() function is defined in this file which is triggered on Button click to open the Alert Box.
Ionic Popup Alerts Example: Script Part
import { Component } from '@angular/core'; import { AlertController } from 'ionic-angular'; @Component({ templateUrl: 'demo.html' }) export class DemoPage { constructor(public atrCtrl: AlertController) { } showAlert() { let alert = this.atrCtrl.create({ title: 'Basic Alert!', subTitle: 'You do not have enough amount in your wallet, Please add more Money!', buttons: ['OK'] }); alert.present(); } } |
Import AlertsController, add in constructor and call create() method with parameters.
So we can see clearly only following things are required to create alerts.
1. importing AlertController.
2. calling it in constructor.
The output of above example will be something like this-
Confirm Alerts | Confirmation Box | Yes | No
Confirmation Alert is used to get acceptance of user. Here is simple Confirmation Box
- 1. HTML Part – It contains the Html Part.
- 2. Script Part – It contains typescript code for the Confirmation Alerts.
Html Part : demo.html
Html template contains button to Call the Confirm Alert which calls showConfirmAlert Function –
Ionic Confirmation Box Alert Example: Html Part
<ion-content padding> <h2>Confirm Alert</h2> <button ion-button block (click)="showConfirmAlert()"> Show Confirm Alert </button> </ion-content> |
Script Part : demo.ts
This contains definition of showConfirmAlert() function which is used to show confirm box –
Ionic Confirmation box Alerts Example: Script Part
import { Component } from '@angular/core'; import { AlertController } from 'ionic-angular'; @Component({ templateUrl: 'demo.html' }) export class DemoPage { constructor(public atrCtrl: AlertController) { } showConfirmAlert() { let alertConfirm = this.atrCtrl.create({ title: 'Delete Items', message: 'Are You Sure to delete this itemss?', buttons: [ { text: 'No', role: 'cancel', handler: () => { console.log('No clicked'); } }, { text: 'Yess', handler: () => { console.log('Yes clicked'); } } ] }); alertConfirm.present(); } } |
The output of above example will look something like this –
Prompt Alerts
This basically used to get user input for example- Enter Wifi password.
- 1. HTML Part – It contains the Html Part.
- 2. Script Part – It contains typescript code for the Prompt Alerts.
Html Part : demo.html
This contains button to Call the Prompt Alert box which calls prompt Function –
Ionic Prompt Alerts Example: Html Part
<ion-content padding> <h2>Prompt Alert</h2> <button ion-button block (click)="showPromptAlert()"> Show Prompt Alert </button> </ion-content> |
Script Part : demo.ts
Script Part contains the definition of prompt function –
Ionic Prompt box Alerts Example: Script Part
import { Component } from '@angular/core'; import { AlertController } from 'ionic-angular'; @Component({ templateUrl: 'demo.html' }) export class DemoPage { constructor(public atrCtrl: AlertController) { } showPromptAlert() { let alert = this.atrCtrl.create({ title: 'Login', inputs: [ { name: 'username', placeholder: 'Enter Username' }, { name: 'password', placeholder: 'Enter Password', type: 'password' } ], buttons: [ { text: 'Cancel', role: 'cancel', handler: data => { console.log('You Clicked on Cancel'); } }, { text: 'Login', handler: data => { if (User.isValid(data.username, data.password)) { // login is valid } else { // invalid login return false; } } } ] }); alert.present(); } } |
The Above Example Will Output something like this –
Radio Alerts
This shows radio options to user and user can select only one option from list.
- 1. HTML Part – It contains the Html Part.
- 2. Script Part – It contains typescript code for the Radio Alerts.
Html Part : demo.html
Html template contains button to which triggers radio alert Function –
Ionic Radio Popup Alert Box Example: Html Part
<ion-content padding> <h2>Radio Alert Demo</h2> <button ion-button block (click)="showRadioAlert()"> Show Radio Alert </button> </ion-content> |
Script Part : demo.ts
It contains function definition
Ionic Radio Alert Popup box Example: Script Part
import { Component } from '@angular/core'; import { AlertController } from 'ionic-angular'; @Component({ templateUrl: 'demo.html' }) export class DemoPage { constructor(public atrCtrl: AlertController) { } showRadioAlert() { let alert = this.atrCtrl.create(); alert.setTitle('Select Gender'); alert.addInput({ type: 'radio', label: 'Male', value: 'Male', checked: true }); alert.addInput({ type: 'radio', label: 'Female', value: 'Female', checked: true }); alert.addButton('Cancel'); alert.addButton({ text: 'OK', handler: data => { this.testRadioOpen = false; this.testRadioResult = data; } }); alert.present(); } } } |
Radio Alert output looks like this
Checkbox Alerts
It is used to provide multiple options and user can select more than one option from list.
- 1. HTML Part – It contains the Html Part.
- 2. Script Part – It contains typescript code for the Checkbox Alerts.
Html Part : demo.html
Html template contains button to Call Checkbox alert Function –
Ionic Checkbox Alerts Box Example: Html Part
<ion-content padding> <h2>Radio Checkbox Demo</h2> <button ion-button block (click)="showCheckboxAlert()"> Show Checkbox Alert </button> </ion-content> |
Script Part : demo.ts
This contains definition of showCheckboxAlert() function –
Ionic Checkbox Alert box Alerts Example: Script Part
import { Component } from '@angular/core'; import { AlertController } from 'ionic-angular'; @Component({ templateUrl: 'demo.html' }) export class DemoPage { constructor(public atrCtrl: AlertController) { } showCheckboxAlert() { let alert = this.atrCtrl.create(); alert.setTitle('Which Fruit do you like?'); alert.addInput({ type: 'checkbox', label: 'Mango', name: 'input-mango', id: 'input-mango', value: 'Mango', checked: true }); alert.addInput({ type: 'checkbox', label: 'Banana', name: 'input-banana', id: 'input-banana', value: 'Banana' }); alert.addInput({ type: 'checkbox', label: 'Apple', name: 'input-apple', id: 'input-apple', value: 'Apple' }); alert.addButton('Cancel'); alert.addButton({ text: 'Okay', handler: data => { console.log('Checkbox data:', data); } }); alert.present(); } } |
Learn More
Don’t Stop! Learn More..
Instance Members
config
create(options)
Display an option with title, inputs and buttons.
-
options – Options to configure the alert box.
Options
Following options are available-
Property | Type | Detail |
---|---|---|
title | string | This is used to add the title for alert box. |
subTitle | string | Adds subtitle for alert box. |
message | string | Adds message for alert box |
cssClass | string | Adds custom css classes, Add classes separated by space. |
inputs | Array | An array of inputs, See input options below. |
buttons | Array | An array of buttons. See options below. |
enableBackdropDismiss | Boolean | Disable backdrop tap dismiss, set false, default value for this option is true. |
Input options
Property | Type | Description |
---|---|---|
type | string |
This is used to define the input type such as – text, email, number, etc. |
name | string |
The name for the input box. |
placeholder | string |
This is used to add the placeholder for input box. |
value | string |
Adds default value of input. |
label | string |
Adds label of input. |
checked | boolean |
Whether or not the input is checked. |
id | string |
Add input box id. |
Button Options
Property | Type | Description |
---|---|---|
text | string |
Adds button text. |
handler | any |
Emitted when the button is pressed. |
cssClass | string |
Adds custom css class to button. |
role | string |
The buttons role, null or cancel. |
Keywords
Ionic popup modal, template, popup close, alert, button style, window etc.
Advertisements
User Ans/Comments
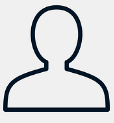
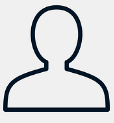
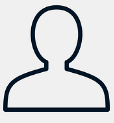
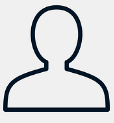
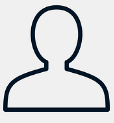